1. Generics allow you to define type-safe data structures, without committing to actual data types.
2. Result higher quality of code by reuse data processing algorithm without duplicating type-specific code.
3. Generics allow you to define type-safe classes without compromising type safety, performance, or productivity.
Example:
.Net 1.1 way of implementing Stack data structure providing Push() and Pop() methods.
For general purpose of Stack, it used to store instances of various type. Old way using Object-based stack, which is amorphous object. This is because Object is canonical .NET base type, it able to hold any type of items.
Problem:
1. Performance in boxing and unboxing value types when pushing and popping process.
2. Type safety. Compiler allow anything cast from Object type. Hence, programmer losing compile-time type safety. Cast wrongly will raise an invalid cast exception at run time.
Hence, to solve this, old way is to create type-specific Stack data structure. For Interger, using InsStack, for String, use StringStack.
Writing type-specific data structures is a tedious, repetitive, and error-prone task.
In addition, there is no way to foresee the use of unknown or yet-undefined future types, so you have to keep an Object-based data structure as well.
Sample Code Download : Learn_Generics.zip
Wednesday, June 30, 2010
Hashtable Vs Arraylist
Arraylist:
1. A Collection of Items containing a Single Item.
2. Can Add any datatype value, every item in arraylist is treated as object.
3. It will dynamically expand and contract when you add/remove elements to it.
Hashtable:
1. Collection of key,value pairs.
2. Key Can be any data-type.
3. Key Cannot be null reference, but value can be.
4. Each element is a key/value pair stored in a DictionaryEntry object.
Retrieving by key in Hashtable is faster than retrieving in Arraylist.
Sample Code Download : Hashtable_Vs_ArrayList.zip
1. A Collection of Items containing a Single Item.
2. Can Add any datatype value, every item in arraylist is treated as object.
3. It will dynamically expand and contract when you add/remove elements to it.
Hashtable:
1. Collection of key,value pairs.
2. Key Can be any data-type.
3. Key Cannot be null reference, but value can be.
4. Each element is a key/value pair stored in a DictionaryEntry object.
Retrieving by key in Hashtable is faster than retrieving in Arraylist.
Sample Code Download : Hashtable_Vs_ArrayList.zip
Tuesday, June 29, 2010
Event Vs Delegate
Event:
An event is a message sent by an object to signal the occurrence of an action. The object that raises the event is called the event sender. The object that captures the event and responds to it is called the event receiver. In event communication, the event sender class does not know which object or method will receive (handle) the events it raises. What is needed is an intermediary (or pointer-like mechanism) between the source and the receiver. The .NET Framework defines a special type (Delegate) that provides the functionality of a function pointer.
Delegate:
A delegate is a class that can hold a reference to a method.
A delegate class has a signature, and it can hold references only to methods that match its signature. Thus equivalent to a type-safe function pointer or a callback.
Sample Code Download : Event-Delegate-Learn.zip
An event is a message sent by an object to signal the occurrence of an action. The object that raises the event is called the event sender. The object that captures the event and responds to it is called the event receiver. In event communication, the event sender class does not know which object or method will receive (handle) the events it raises. What is needed is an intermediary (or pointer-like mechanism) between the source and the receiver. The .NET Framework defines a special type (Delegate) that provides the functionality of a function pointer.
Delegate:
A delegate is a class that can hold a reference to a method.
A delegate class has a signature, and it can hold references only to methods that match its signature. Thus equivalent to a type-safe function pointer or a callback.
Sample Code Download : Event-Delegate-Learn.zip
Different Between Abstract Method And Virtual Method
Abstract method vs Virtual methods
Abstract method: When a class contains an abstract method, that class must be declared as abstract. The abstract method has no implementation and thus, classes that derive from that abstract class, must provide an implementation for this abstract method.
A subclass which derives from an abstract class and fails to implement abstract methods will fail to compile.
Virtual method: A class can have a virtual method. The virtual method has an implementation. When you inherit from a class that has a virtual method, you can override the virtual method and provide additional logic, or replace the logic with your own implementation.
Virtual methods allow subclasses to provide their own implementation of that method using the override keyword.
A member defined as virtual must be implemented in the base class, but may be optionally overridden in the derived lass if different behavior is required.
Sample Code Download : Learn_Abstract_Vs_Virtual.zip
Abstract method: When a class contains an abstract method, that class must be declared as abstract. The abstract method has no implementation and thus, classes that derive from that abstract class, must provide an implementation for this abstract method.
A subclass which derives from an abstract class and fails to implement abstract methods will fail to compile.
Virtual method: A class can have a virtual method. The virtual method has an implementation. When you inherit from a class that has a virtual method, you can override the virtual method and provide additional logic, or replace the logic with your own implementation.
Virtual methods allow subclasses to provide their own implementation of that method using the override keyword.
A member defined as virtual must be implemented in the base class, but may be optionally overridden in the derived lass if different behavior is required.
Sample Code Download : Learn_Abstract_Vs_Virtual.zip
What Is The Different Between Abstract Class And Interface
Abstract Base Vs Interface
Abstract class:
An Abstract class without any implementation just looks like an Interface; however there are lot of differences than similarities between an Abstract class and an Interface.
Interface concept:
An interface is not a class.
An interface has no implementation; it only has the signature.
It just the definition of the methods without the body.
Similarity between Abstract class and Interface:
They act as a contract that is used to define hierarchies for all their subclasses.
Different:
A class can implement more than one interface but can only inherit from one abstract class.
Since C# doesn't support multiple inheritance, interfaces are used to implement multiple inheritance.
Sample Code Download: AbstractBase_Vs_Interface.zip
Abstract class:
An Abstract class without any implementation just looks like an Interface; however there are lot of differences than similarities between an Abstract class and an Interface.
- We cannot make instance of abstract class.
- An abstract class is only to be sub-classed (inherited from).
- In other words, it only allows other classes to inherit from it but cannot be instantiated.
Interface concept:
An interface is not a class.
An interface has no implementation; it only has the signature.
It just the definition of the methods without the body.
Similarity between Abstract class and Interface:
They act as a contract that is used to define hierarchies for all their subclasses.
Different:
A class can implement more than one interface but can only inherit from one abstract class.
Since C# doesn't support multiple inheritance, interfaces are used to implement multiple inheritance.
Sample Code Download: AbstractBase_Vs_Interface.zip
Monday, June 28, 2010
Interview Question: Will Code In Finally Block Being Executed When There Is A 'Return' In Try Block
Will Code In Finally Block Being Executed When There Is A 'Return' In Try Block?
Finally block allow us to specify code that's guaranteed to execute no matter what kind of exception a thread throws.
Sample Code: Try_Catch_Finally.zip
Finally block allow us to specify code that's guaranteed to execute no matter what kind of exception a thread throws.
Sample Code: Try_Catch_Finally.zip
Subscribe to:
Posts (Atom)
Image Understanding (IU)
Throughout these years, the growth of digital media collections has been accelerating, particularly in still images. These artifacts repre...
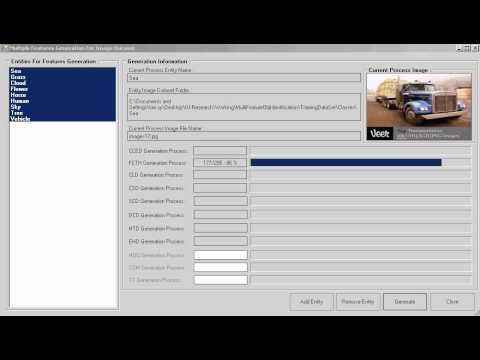
-
Year: 2001 Programming Language: Java Source Code: Email the author: ahyeek@gmail.com Application For Test: ahyeek@gmail.com Related Doc...
-
Year: 2004 - 2005 Programming Language: OpenGL, C, C++, Titanium Award: Gold medal award of the Invention Exhibition of New Invention,...
-
Year: 2005 - 2006 Programming Language & Tools: Microsoft .NET C# Source code / Request for customization: http://www.geneticalgorithm....